Kubernetes has become the de facto container orchestration platform for running and scaling application workloads. When deploying the application on Kubernetes, some specific commands might need to be run in the container to ensure that it is running properly or has the desired configurations. You might even need to run some commands within a sidecar container to generate and store log data.
This blog will examine how commands and arguments can be used when creating Kubernetes pods with some hands-on examples. Adding commands to Docker containers can be done using CMD and ENTRYPOINT while creating the Dockerfile. If you are unfamiliar with how this works in Docker, please refer to this blog, as understanding commands in Kubernetes is a prerequisite.
What are commands & Arguments in Kubernetes?
Before we move on to the actual differences between commands and arguments, let’s first try and understand what exactly they are and why we require them. Both parameters are used to pass certain parameters to the container running within the pod. If you are familiar with commands and arguments within bash or any shell environment, this will be easy to understand.
- Command: A piece of executable code that is run within the container shell
- Argument: Additional inputs for the command to execute a certain behavior
Let’s try and understand this with an example. Consider the following bash command:
echo “Hello world”
You may be familiar with the above command. It simply prints out the string Hello World. In the above example, echo is the command, and "Hello World" is the argument.
This is a similar functioning within Kubernetes. If we try to relate the Kubernetes way of defining commands and arguments with how it is defined in Docker, it can be thought of in the following way:
Kubernetes | Docker |
---|---|
Command | Entrypoint |
Args | CMD |
You might be wondering, if commands are already defined while creating the Docker containers, wouldn’t it conflict if you try to define them within Kubernetes? The answer to this is no.
When you use the command
and arguments
fields in the pod’s definition file, it will override the commands that were used when building the container.
Similarly, if you do not pass in any values in the pod’s definition file, then by default, the commands that were defined within the container will be used.
However, when you create the Docker container, you can change the values of CMD and Entrypoint by passing in appropriate flags in the docker run
command. This is not possible within Kubernetes.
Once the pod is created and is running, the commands cannot be edited. If you need to edit the commands, you must recreate the pod.
Kubernetes Commands
As we have already discussed earlier, a command is the executed piece of code that we run within the container. Not to confuse it with the application code, commands are separate system binaries that exist within the container.
If you run a minimal custom container, you will first need to ensure that the package exists within the container.
This is similar to running commands in your local system. For example, you will not be able to run `kubectl if you have not installed the correct package. This same behavior is true for the container as well.
Let’s create a simple nginx pod and add a command to it for printing "Hello World"
:
apiVersion: v1
kind: Pod
metadata:
name: nginx-hello
spec:
containers:
- image: nginx
name: nginx
command: ["echo","Hello World"]
To check if this command has worked or not, you can check the logs of this pod.
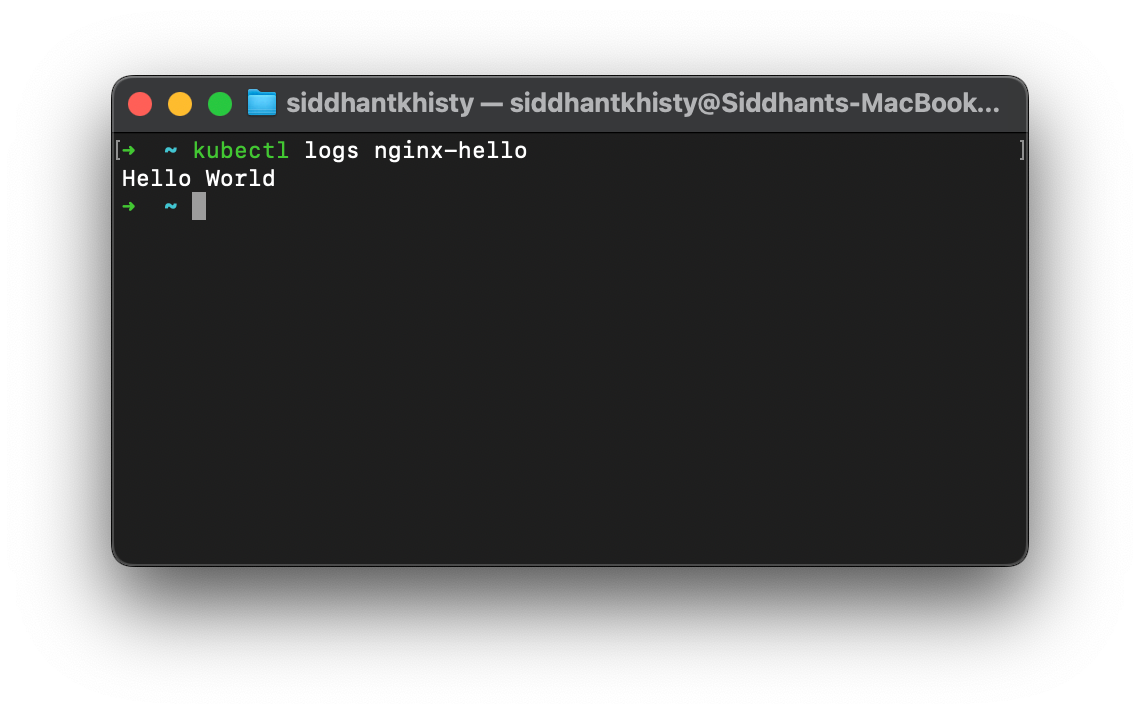
You also have the option to define the commands as an array within YAML:
apiVersion: v1
kind: Pod
metadata:
name: nginx-hello
spec:
containers:
- image: nginx
name: pod
command:
- echo
- Hello
- World
You can quickly create a pod using imperative commands:
kubectl run pod –image=nginx –command – echo “hello world”
In the above example, echo hello world
will be interpreted as a command and will appear as an array in the created YAML.
If you do not specify — command, it will be read as arguments.
To preview YAML without creating the resource:
kubectl run pod --image=nginx --dry-run=client -o yaml --command – echo “hello world”
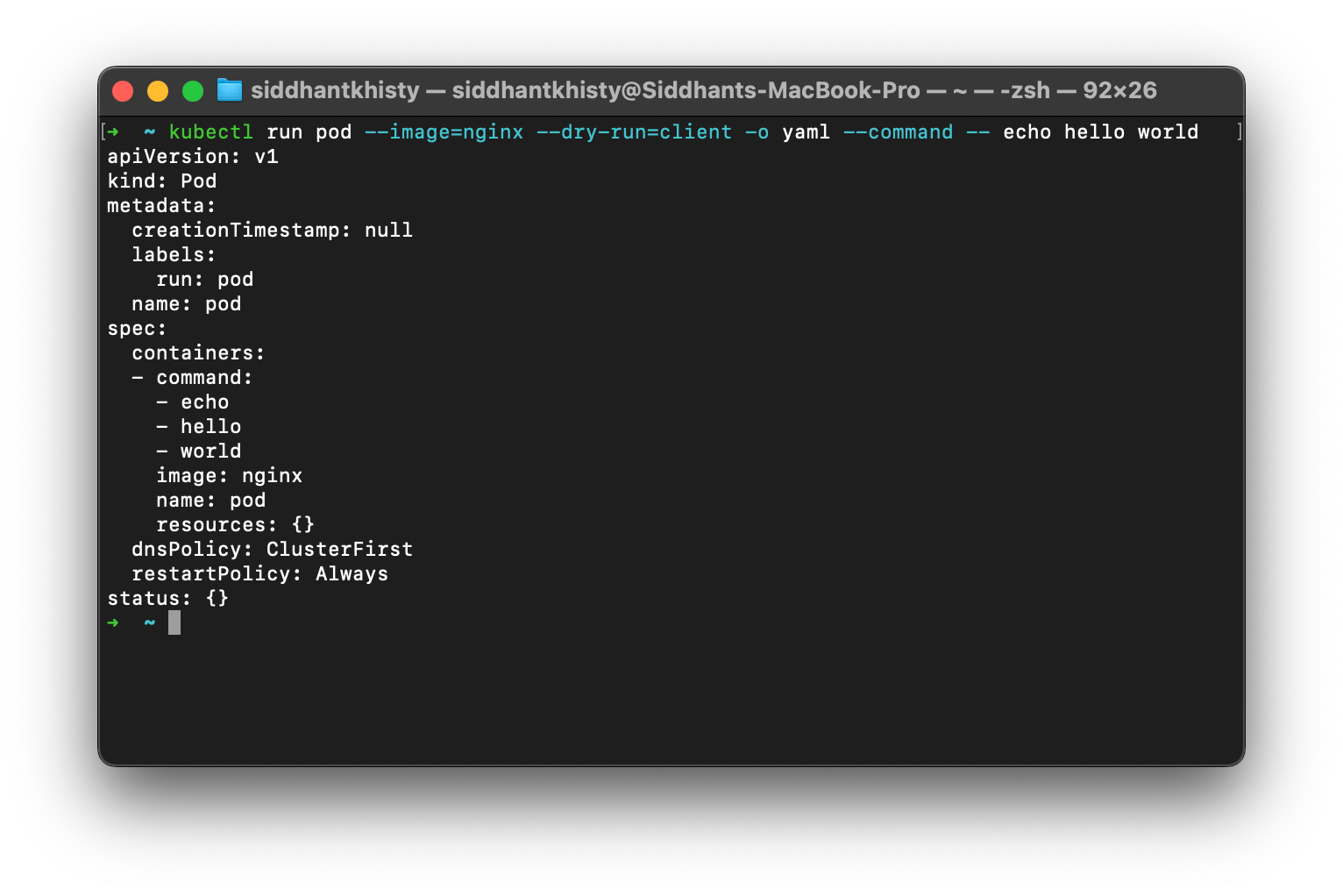
Kubernetes Arguments
Arguments in Kubernetes are the additional inputs that come after the command. They provide instructions on the behavior that should occur when the container is created and the commands are run.
Just like commands, arguments cannot be changed once the pod is running, and you must recreate the pod.
Example using declarative YAML:
apiVersion: v1
kind: Pod
metadata:
name: busybox-sleeper
spec:
containers:
- args:
- sleep
- "5000"
image: busybox
name: busybox-sleeper
The above YAML file creates a pod with the busybox container, and put’s it to sleep. The default behavior of the busybox container is to exit out as soon as it’s process is completed. We can infer that the sleep command is running, as the pod is in the running state.
Note: In the below images, I have used an aliased kubectl to k. If you wish to set the name, you can run the below command
echo “alias k=kubectl” >> ~/.bashrc
source ~/.bashrc
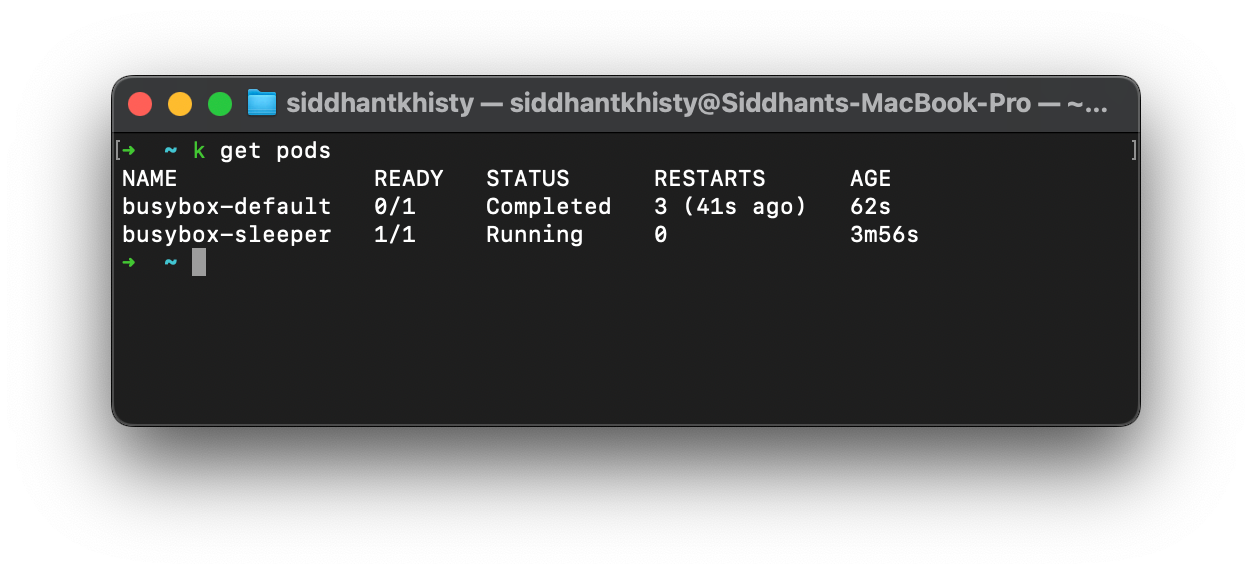
Imperative version:
kubectl run busybox-sleeper --image=busybox -- sleep 5000
To preview the YAML:
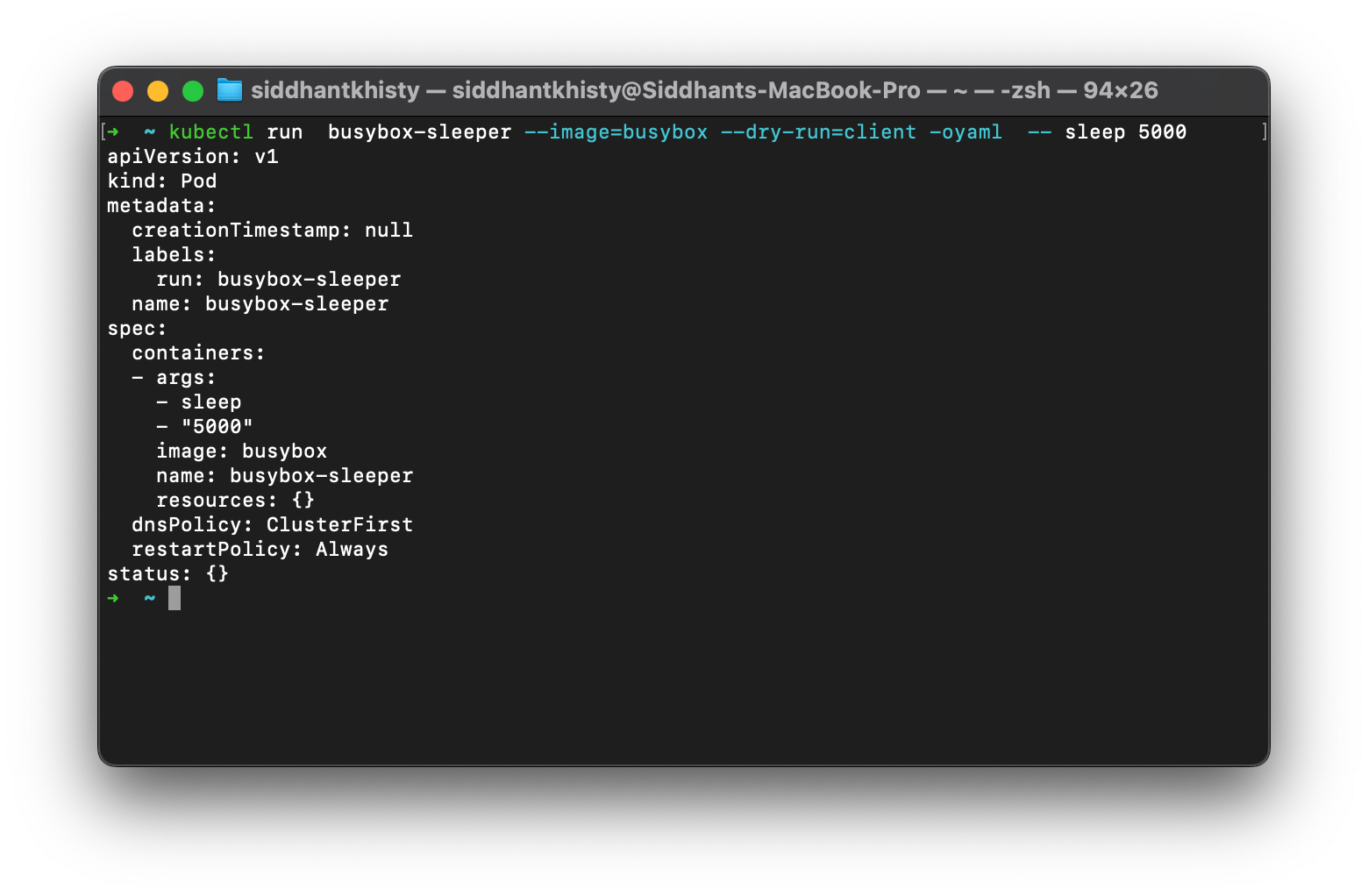
Even when the command field is omitted and only args are passed, the container behaves correctly. This is due to how the shell interprets inputs—Kubernetes replicates that behavior.
Combining Kubernetes Commands and Arguments
While commands and arguments can sometimes work interchangeably, best practice is to use both.
Example using both:
apiVersion: v1
kind: Pod
metadata:
name: busybox-combine
spec:
containers:
- name: busybox
image: busybox
command: ["echo"]
args: ["hello", "world"]
For multiple commands, use shell syntax with command: ["/bin/sh"] and pass script via args:
apiVersion: v1
kind: Pod
metadata:
name: busybox-multi-cmd
spec:
containers:
- name: busybox
image: busybox
command: ["/bin/sh"]
args: ["-c", "while true; do echo hello; sleep 10;done"]
We can see that this script is being executed and the command is being run every 10 seconds.
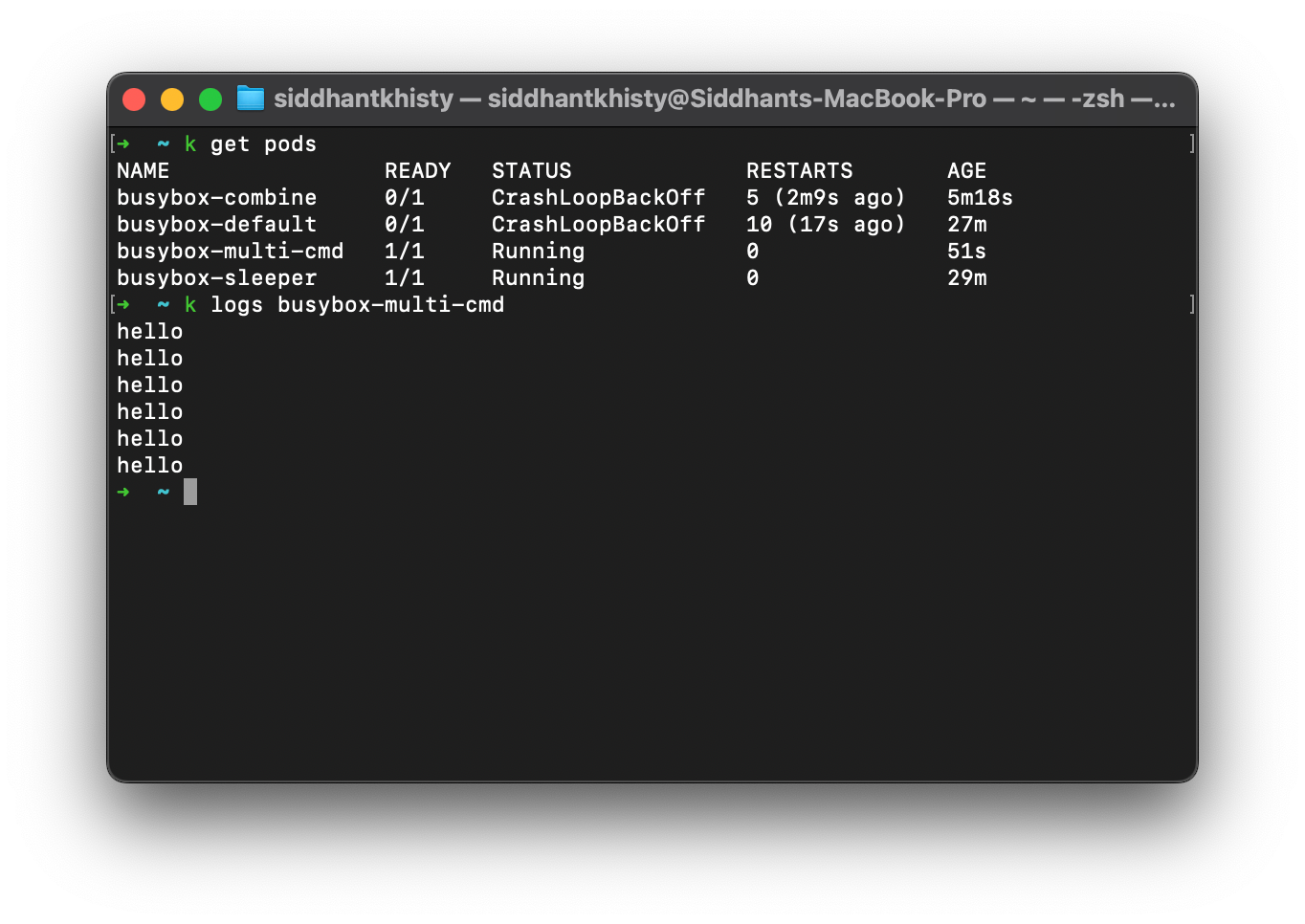
Use Cases for commands & args
You might want to override container commands in scenarios like:
- Running container startup scripts
- Running scripts within an Init container
- Sidecar container generating logs
- Jobs/CronJobs
- Populating databases
- Network testing from inside a pod
Example: ephemeral pod for DNS check
kubectl run network-test --image=busybox --rm -it --restart=Never – nslookup github.com
kubectl run network-test –image=busybox –rm -it –restart=Never – nslookup github.com
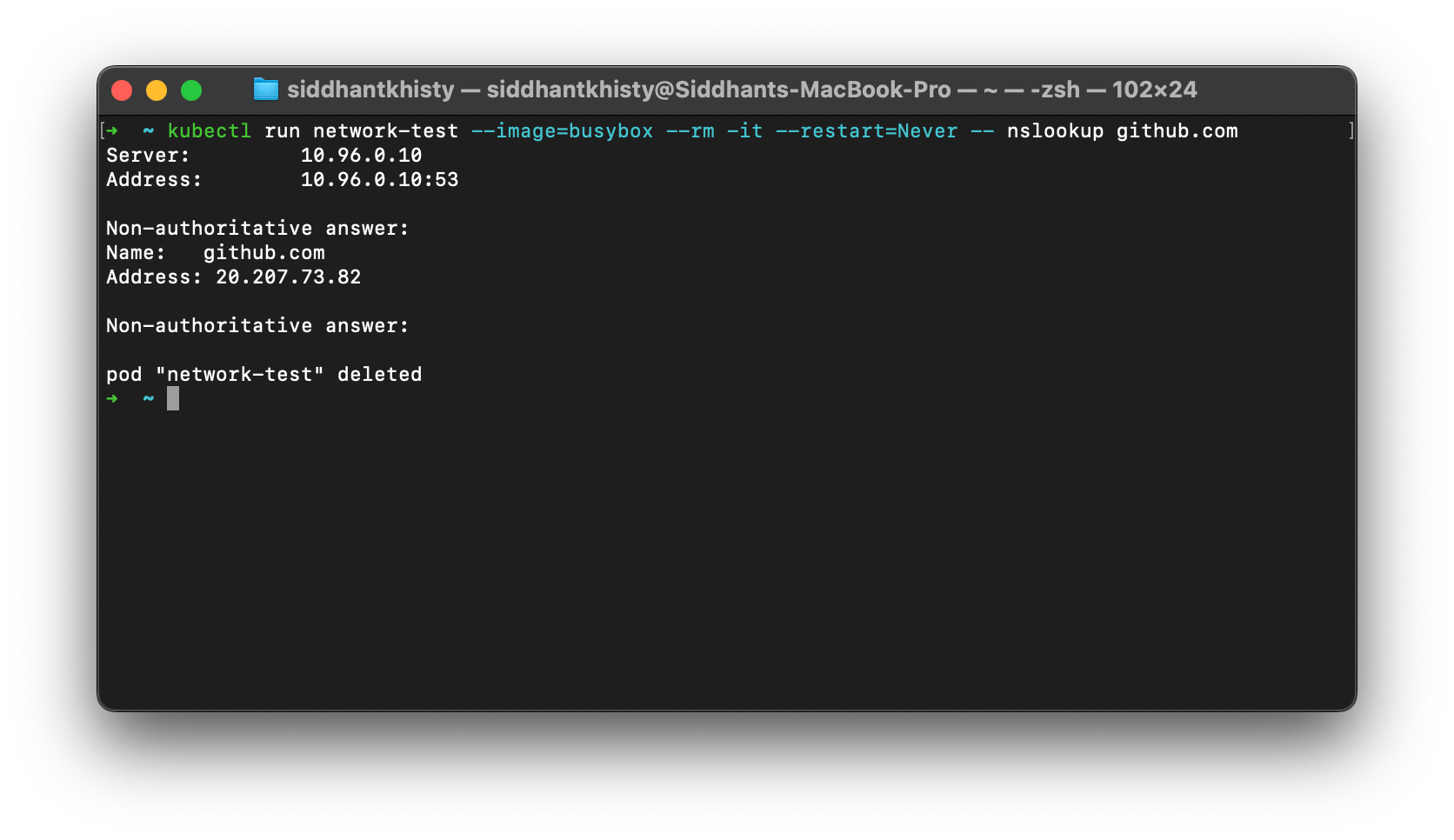
Using Commands & Arguments in Devtron
Devtron is an open-source Kubernetes dashboard aimed at simplifying application lifecycle management.
Devtron provides a base deployment template where you can easily write the required commands and arguments without editing YAML manually.
[Fig. 7] Create Commands & Args in Devtron
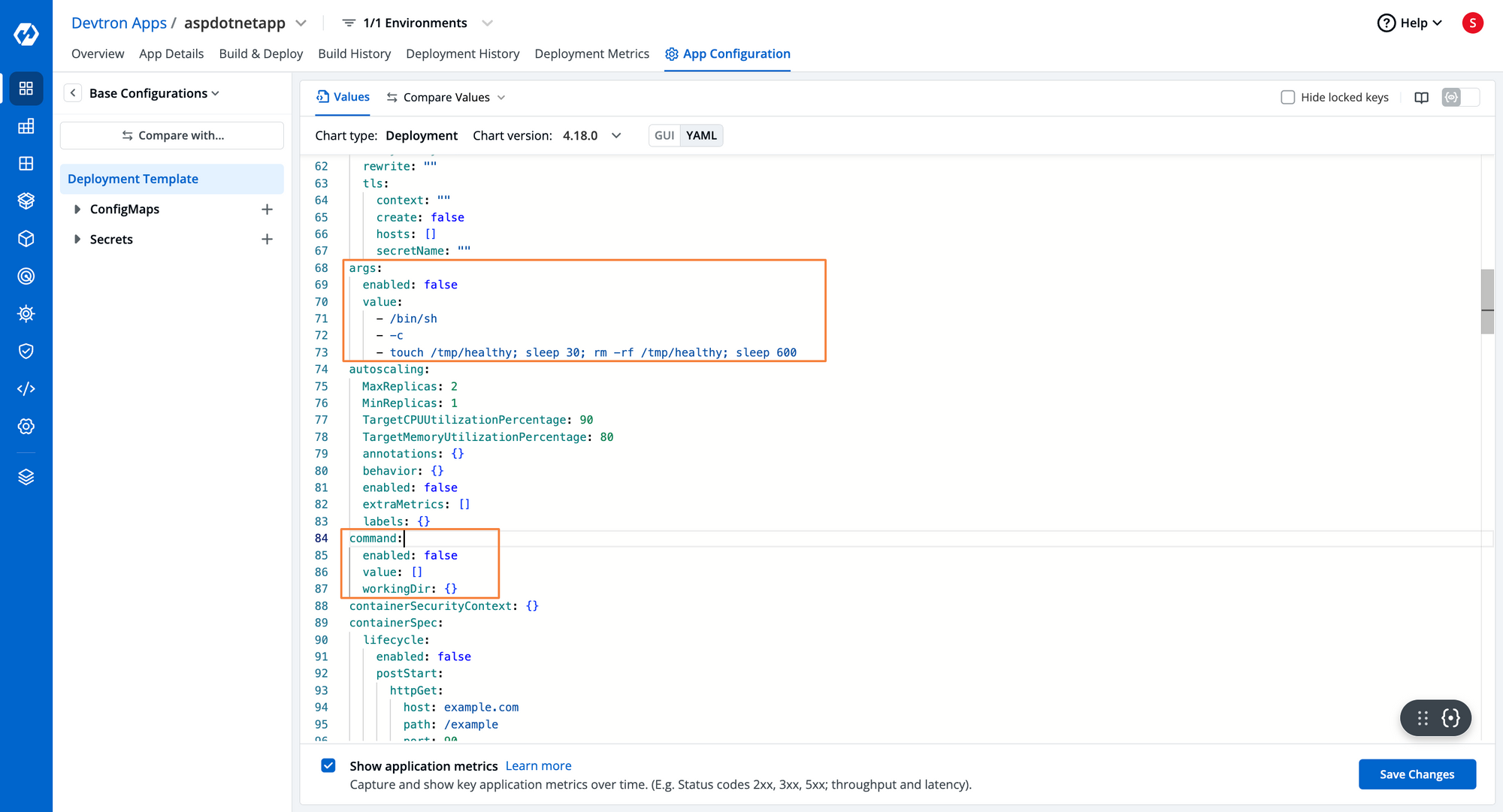
Devtron also supports:
- Rolling back deployments
- Security scans with policies
- Grouping Helm charts
- Visibility into workloads
If you’re looking for a tool to ease the pains of application management, do check out Devtron.
Conclusion
Defining commands to execute within the container is crucial for running containerized workloads without any hassle.
While Docker lets you define or override commands using docker run
, Kubernetes gives you the command and args fields in the pod manifest for that purpose.
If you have any questions regarding how to use these, please join our Discord Community—we’d be happy to help.