Express.js is a minimalist web framework for Node.js, widely regarded as one of the most popular choices for building web applications and APIs. Known for its simplicity and flexibility, Express.js provides a powerful set of features, including robust routing, middleware integration, and support for template engines. Its lightweight design allows developers to create server-side applications with ease, offering the freedom to structure projects without enforcing rigid conventions. Thus, it is a go-to solution for both beginners and experienced developers.
This blog will walk you through the journey of modernizing your Express.js applications for Kubernetes deployment. Whether you're running a high-traffic API service, a microservices architecture, or a traditional web application, you'll learn how to leverage Kubernetes to ensure your Express.js applications are scalable, reliable, and easy to maintain.
We will explore two methods for deploying Express.js applications:
- Using Devtron for Automated Deployment
- Using Kubernetes Manually
Deploying Express.js Applications on Kubernetes
Deploying an Express.js application to Kubernetes involves several steps. Let’s first review the overall process and then discuss the various steps in depth.
Steps for Deployment
- Write and build the Express.js Application
- Containerize the Express.js Application
- Push the container to a Container Registry such as DockerHub
- Create the required YAML Manifest for Kubernetes Resources
- Apply the YAML manifest to the Kubernetes clusters
Prerequisites
Before proceeding with the deployment process, please make sure that you have the following prerequisites
- An Express.js application (You can clone this repo)
- Docker
- Kubectl
- A Kubernetes Cluster such as kind
Method 1: Deploying Express.js Applications Using Devtron
Devtron is a Kubernetes management platform that simplifies the entire DevOps lifecycle. It automates the creation of Dockerfiles, and Kubernetes manifests, builds the application, and manages deployment through an intuitive UI.
Step 1: Create a Devtron Application and Add Git Repository
- From Devtron’s home page, create a new Devtron application.
- Add the Git Repository containing the Express.js application code.
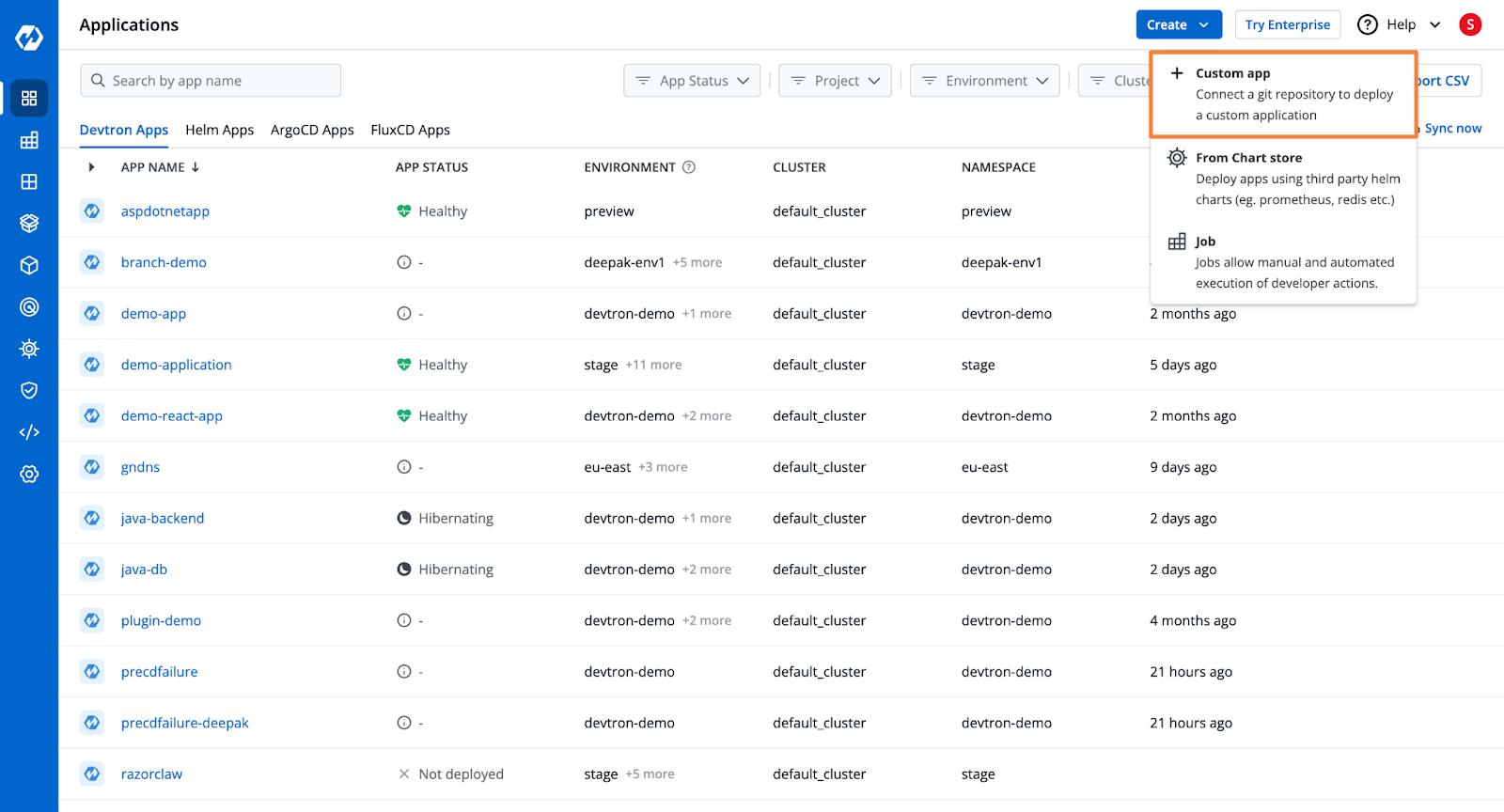
Check out the documentation to learn more about the application creation process.
Step 2: Configure the Build
- Devtron will pull code from the repository and build the Docker container.
- You need to configure an OCI Container Registry.
- Choose from three build options:
- Use an existing Dockerfile
- Create a Dockerfile (using Devtron's template for Express.js applications)
- Use Buildpacks
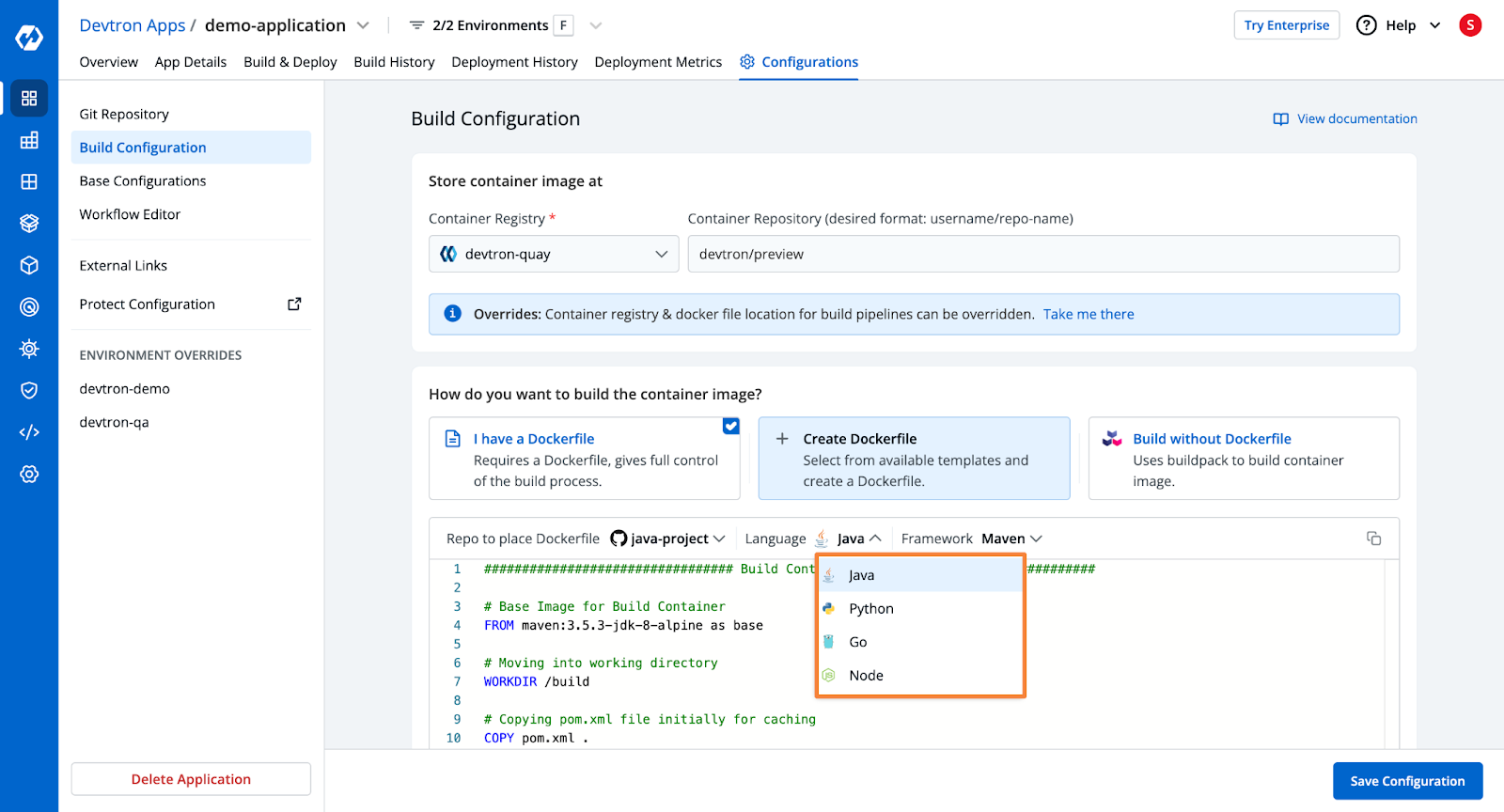
Step 3: Deployment Configurations
- Devtron provides a pre-configured YAML template for Kubernetes deployment.
- Configure ingress, autoscalers, and other deployment settings.
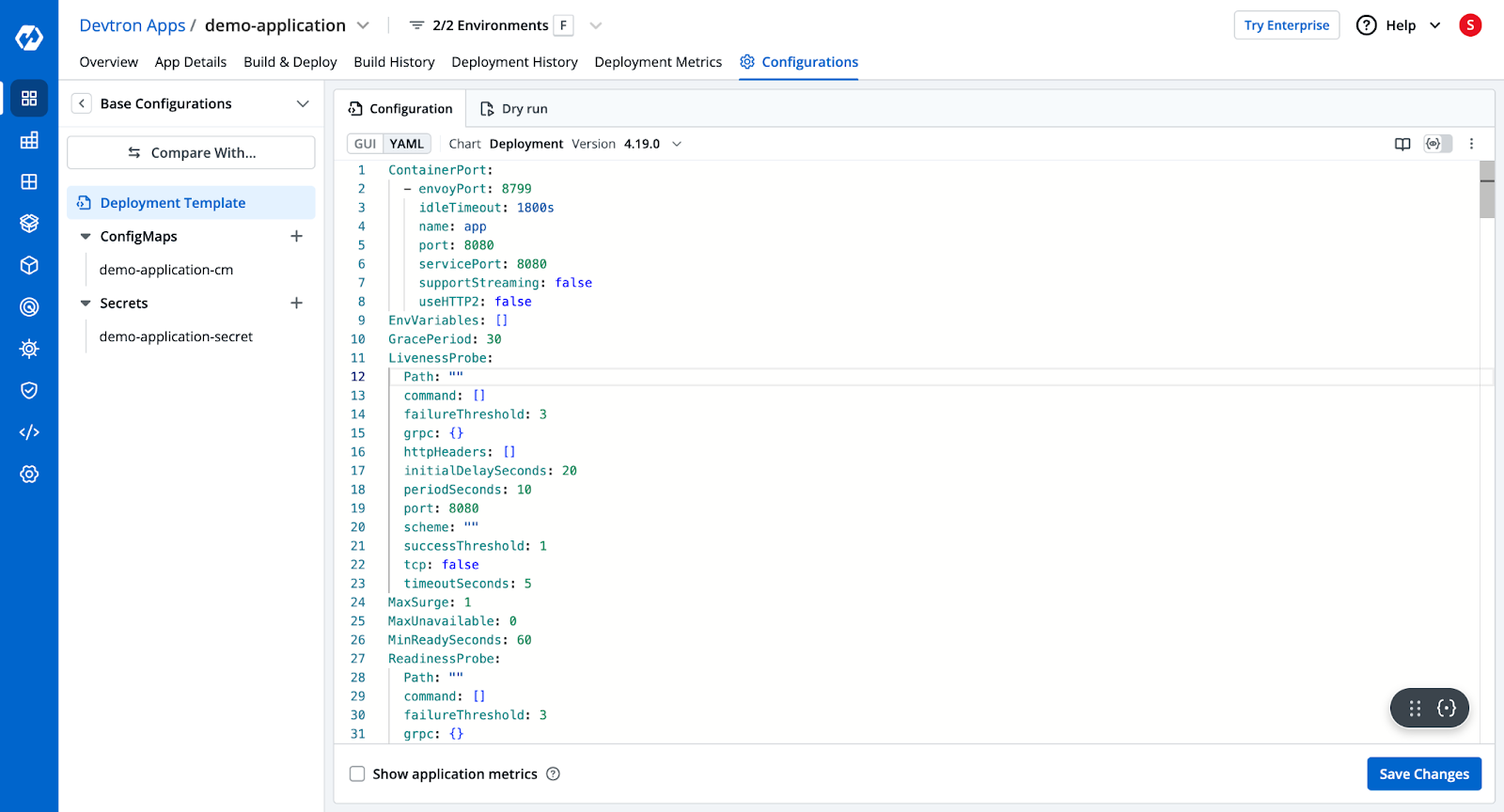
Step 4: Create the CI/CD Pipelines
- The CI pipeline will build the application and push the image to a registry.
- The CD pipeline will trigger deployments in the Kubernetes cluster.
- Configure Pre and Post Stages (e.g., security scanning, unit testing).
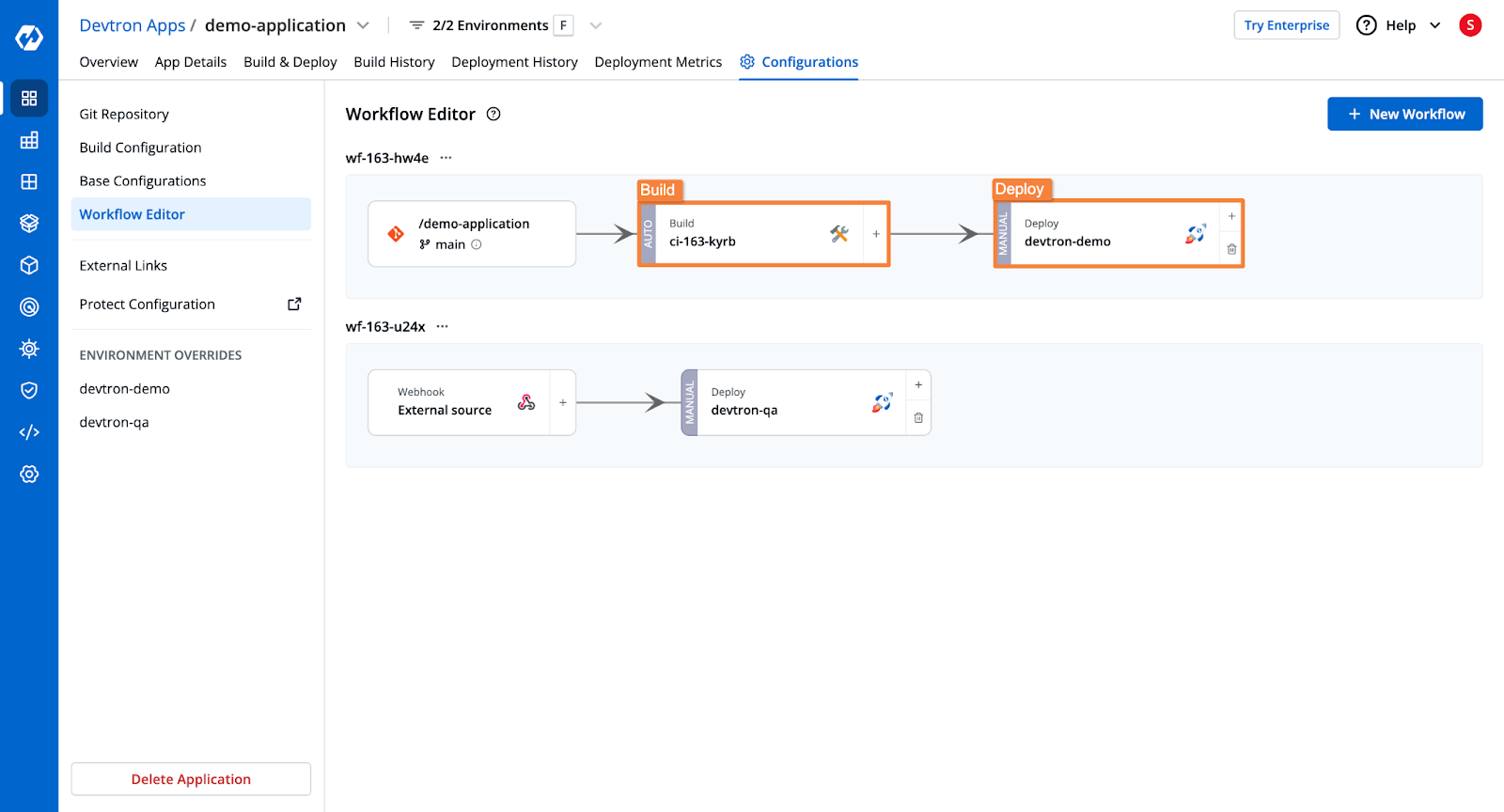
Please check the documentation to learn more about the pipeline configurations.
Step 5: Trigger the Build and Deploy Pipelines
- Select the Git branch and trigger the build stage.
- Once the build is complete, trigger the deployment stage.
- Devtron will deploy the application and show:
- Deployment status
- Application health
- Kubernetes resource details
- Security vulnerabilities
- Rollback options in case of errors
Once the application is deployed, you will be able to see the application's health, deployment status, security vulnerabilities, the Kubernetes resources of the application, and more.
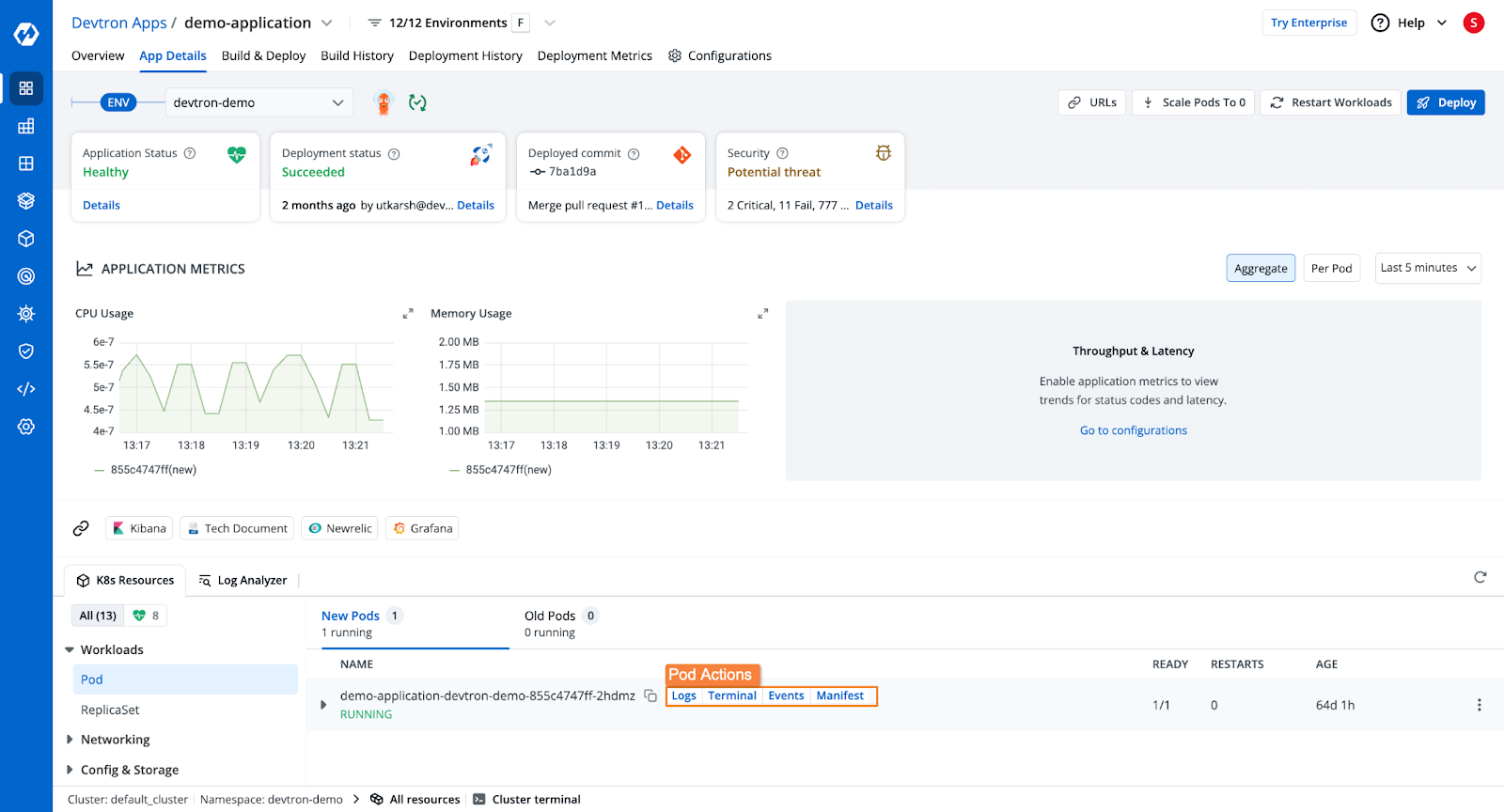
Method 2: Deploying Go Applications Manually To Kubernetes
Step 1: Create the Dockerfile
A Dockerfile is a set of instructions for building a container image. Below is the Dockerfile to containerize your Express.js application:
FROM node
ENV TINI_VERSION v0.18.0
RUN arch=$(arch | sed s/aarch64/arm64/ | sed s/x86_64/amd64/) && echo $arch && wget
https://github.com/krallin/tini/releases/download/${TINI_VERSION}/tini-${arch} -O /tini
RUN chmod +x /tini
ENTRYPOINT ["/tini", "--"]
COPY /. .
RUN npm install
CMD ["node","app.js"]
Step 2: Build and Push the Docker Image
- Run the following command to build the Docker image:
docker build -t devtron/express.js-app:v1 .
- Push the image to DockerHub:
docker push devtron/express.js-app
Step 3: Creating the Kubernetes Deployment and Service Manifests
- Create a deployment.yaml file:
apiVersion: apps/v1
kind: Deployment
metadata:
name: expressnodejs-deployment
spec:
replicas: 3
selector:
matchLabels:
app: express-node
template:
metadata:
labels:
app: express-node
spec:
containers:
- name: node-container
image: bhushannemade/express-node
ports:
- containerPort: 8080
- Create a service.yaml file:
apiVersion: v1
kind: Service
metadata:
name: express-node-service
spec:
selector:
app: express-node
ports:
- protocol: TCP
port: 80
targetPort: 8080
type: NodePort
Step 4: Deploy to Kubernetes
- Run the following command to apply the manifests:
kubectl apply -f deployment.yaml service.yaml
Your Express.js application has now been deployed to Kubernetes!
Common Challenges and Solutions
1. Container Image Size Management
- Use Multi-Stage Builds to separate build and runtime environments.
- Use Lightweight Base Images like Alpine or Distroless to reduce size.
2. Resource Management
- Set Memory and CPU Limits to avoid overconsumption.
- Implement Autoscaling (HPA) to handle varying workloads.
3. Deployment Strategies
- Rolling Updates to ensure zero-downtime deployments.
- Graceful Shutdown Handling to avoid breaking live traffic.
Conclusion
In this blog, we explored two approaches for deploying Express.js applications on Kubernetes:
- Manual Kubernetes Deployment using Docker and YAML manifests.
- Automated Devtron Deployment with built-in CI/CD pipelines and advanced configurations.
Using Devtron simplifies Kubernetes deployments, reducing manual efforts and improving efficiency. Start deploying applications today using Devtron’s platform!
FAQ
How to deploy an Express.js application on Kubernetes?
Deploying an Express.js application on Kubernetes involves creating a Docker image of the app, preparing Kubernetes manifests (for deployment and service), pushing the image to a container registry, and applying the manifests to a Kubernetes cluster.
What is the role of Kubernetes in deploying Express.js applications?
Kubernetes automates the deployment, scaling, and management of Express.js applications. It ensures reliability, performance, and scalability, especially in cloud-native environments.
What is Devtron and how does it simplify Express.js deployments on Kubernetes?
Devtron is an open-source platform that simplifies Kubernetes operations, automating the build and deployment process for Express.js applications, and offering GitOps-enabled CI/CD pipelines for more efficient and reliable deployments.
How to containerize an Express.js app for Kubernetes?
To containerize an Express.js app, create a Dockerfile that installs dependencies, copies application files, and sets the app to run inside a container. Use docker build to create a Docker image.
What tools can automate Express.js deployment on Kubernetes?
Tools like Helm, Jenkins, GitHub Actions, ArgoCD, and FluxCD help automate the deployment of Express.js applications on Kubernetes through CI/CD pipelines.