Laravel is an open-source PHP framework for developing web applications. It follows the model-view-controller (MVC) architectural pattern. It provides an expressive syntax and a robust set of tools and features designed to make development quick and easier while reducing common tasks used in many web projects.
In today's world where pace and reliability need to go hand in hand production environments demand robust capabilities including automated failure handling, self-healing mechanisms, and dynamic scaling to meet varying workloads. These features are essential for maintaining reliable, high-performance applications in real-world scenarios.
Containerizing your legacy Laravel-PHP applications makes them portable and infrastructure-agnostic. The containers package the application, its dependencies, and its runtime environment into standardized units. When combined with Kubernetes orchestration capabilities, this containerization approach enables efficient deployment, scaling, and management of Laravel applications in modern cloud infrastructure.
This blog will walk you through the journey of modernizing your Laravel-PHP applications for Kubernetes deployment. Whether you're running a high-traffic API service, a microservices architecture, or a traditional web application, you'll learn how to leverage Kubernetes to ensure your Laravel applications are scalable, reliable, and easy to maintain.
We will explore two methods for deploying Express.js applications:
- Using Devtron for Automated Deployment
- Using Kubernetes Manually
Deploying PHP-Laravel Applications on Kubernetes
Deploying a PHP-Laravel application to Kubernetes involves several steps. Let’s first review the overall process and then discuss the various steps in depth.
Steps for Deployment
- Write and build the PHP-Laravel Application
- Containerize the PHP-Laravel Application
- Push the container to a Container Registry such as DockerHub
- Create the required YAML Manifest for Kubernetes Resources
- Apply the YAML manifest to the Kubernetes clusters
Prerequisites
Before proceeding with the deployment process, please make sure that you have the following prerequisites
- A PHP-Laravel application
- Docker
- Kubectl
- A Kubernetes Cluster such as kind
Method 1: Deploying PHP-Laravel Applications Using Devtron
Devtron is a Kubernetes management platform that simplifies the entire DevOps lifecycle. It automates the creation of Dockerfiles, and Kubernetes manifests, builds the application, and manages deployment through an intuitive UI.
Step 1: Create a Devtron Application and Add Git Repository
- From Devtron’s home page, create a new Devtron application.
- Add the Git Repository containing the PHP-Laravel application code.
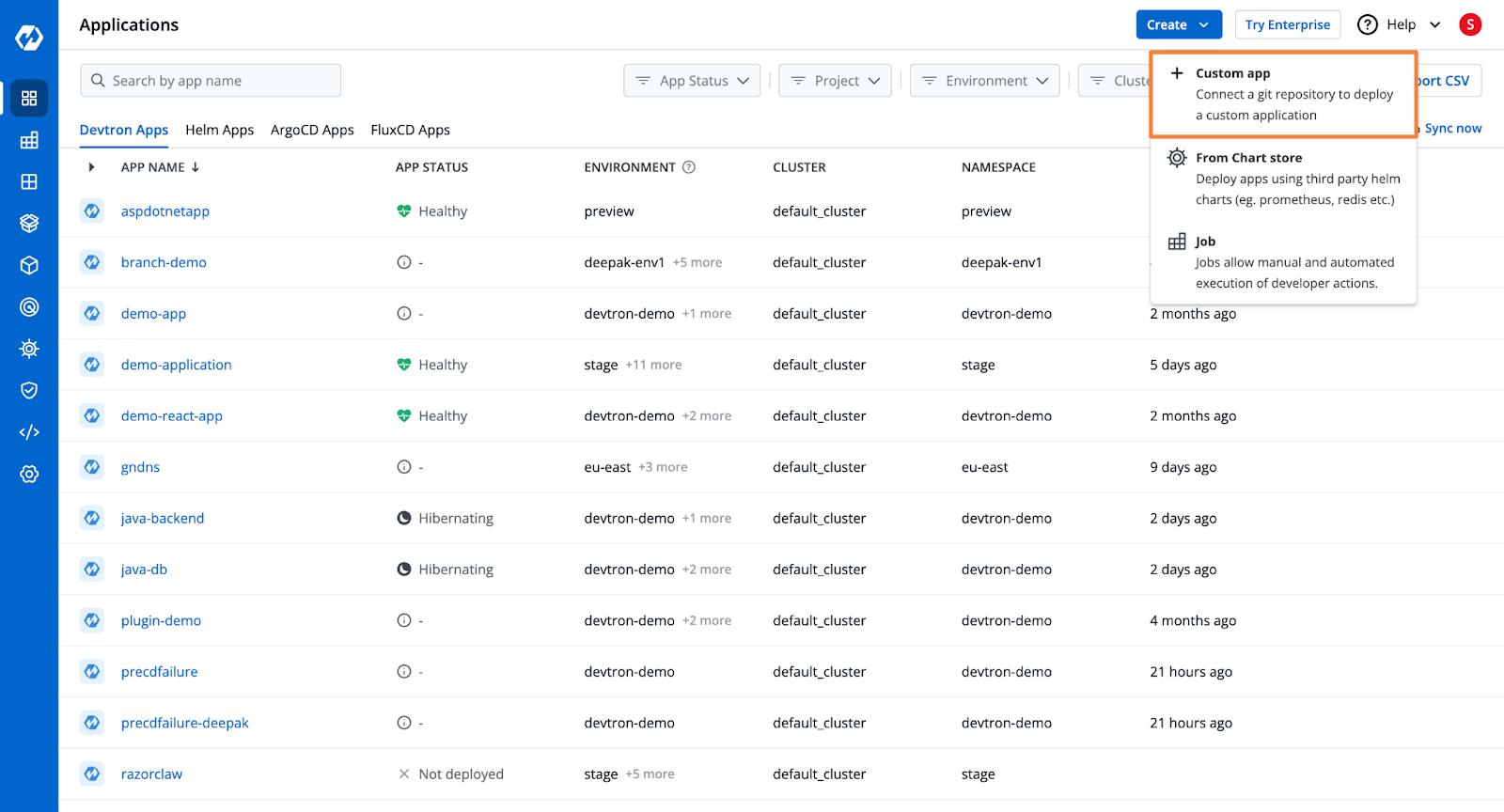
Check out the documentation to learn more about the application creation process.
Step 2: Configure the Build
- Devtron will pull code from the repository and build the Docker container.
- You need to configure an OCI Container Registry.
- Choose from three build options:
- Use an existing Dockerfile
- Create a Dockerfile (using Devtron's template for Go applications)
- Use Buildpacks
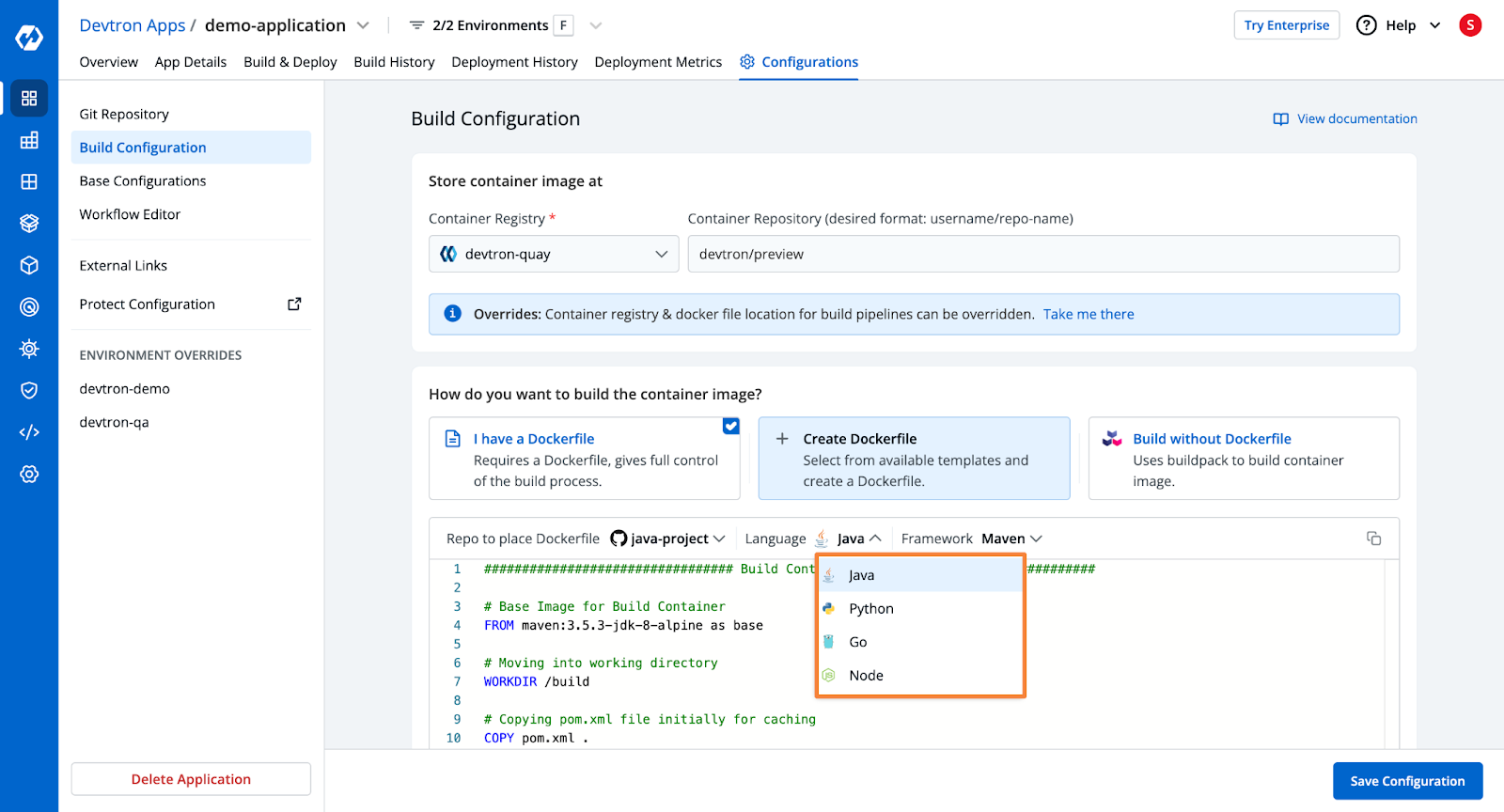
Step 3: Deployment Configurations
- Devtron provides a pre-configured YAML template for Kubernetes deployment.
- Configure ingress, autoscalers, and other deployment settings.
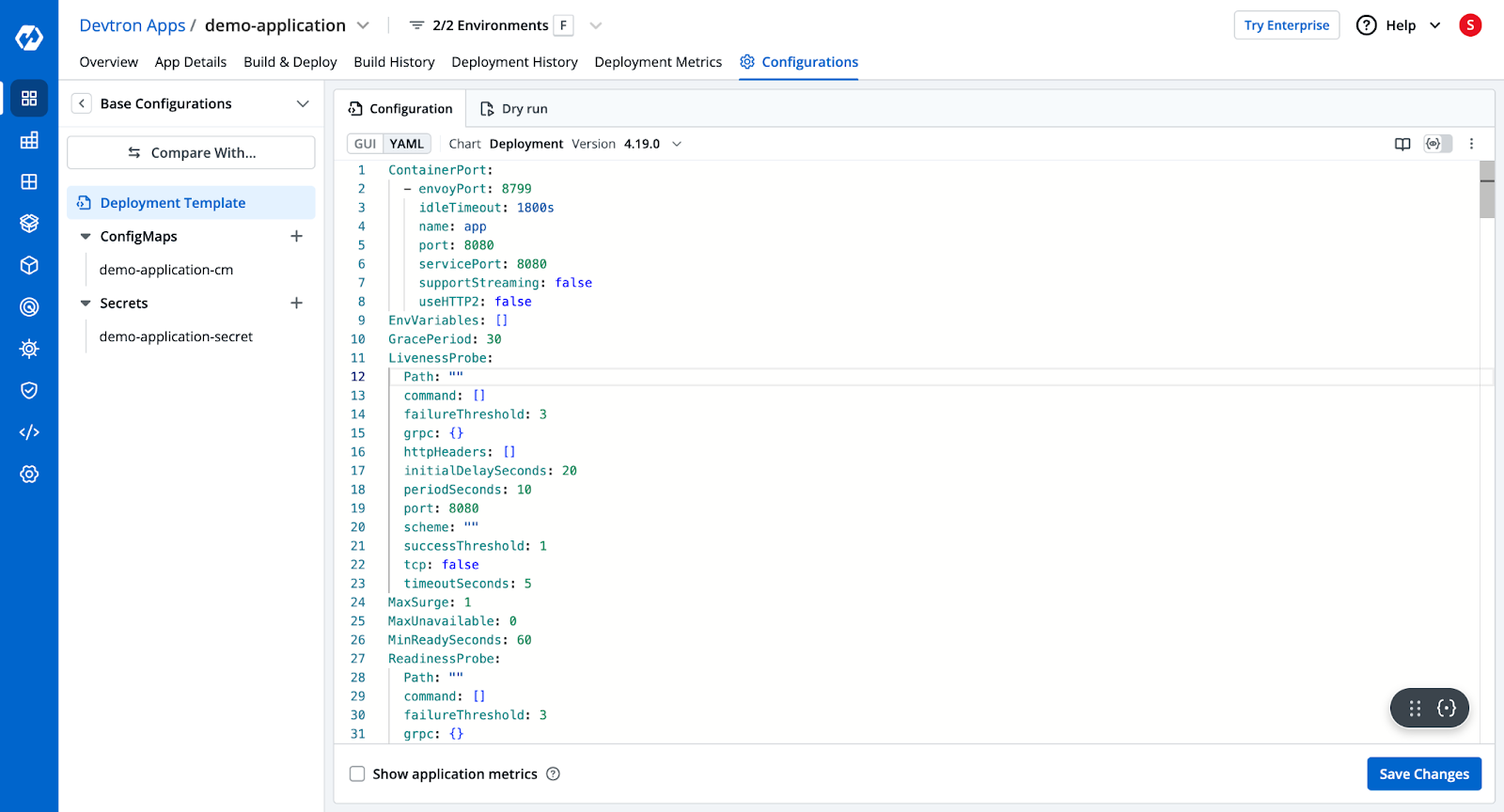
Step 4: Create the CI/CD Pipelines
- The CI pipeline will build the application and push the image to a registry.
- The CD pipeline will trigger deployments in the Kubernetes cluster.
- Configure Pre and Post Stages (e.g., security scanning, unit testing).
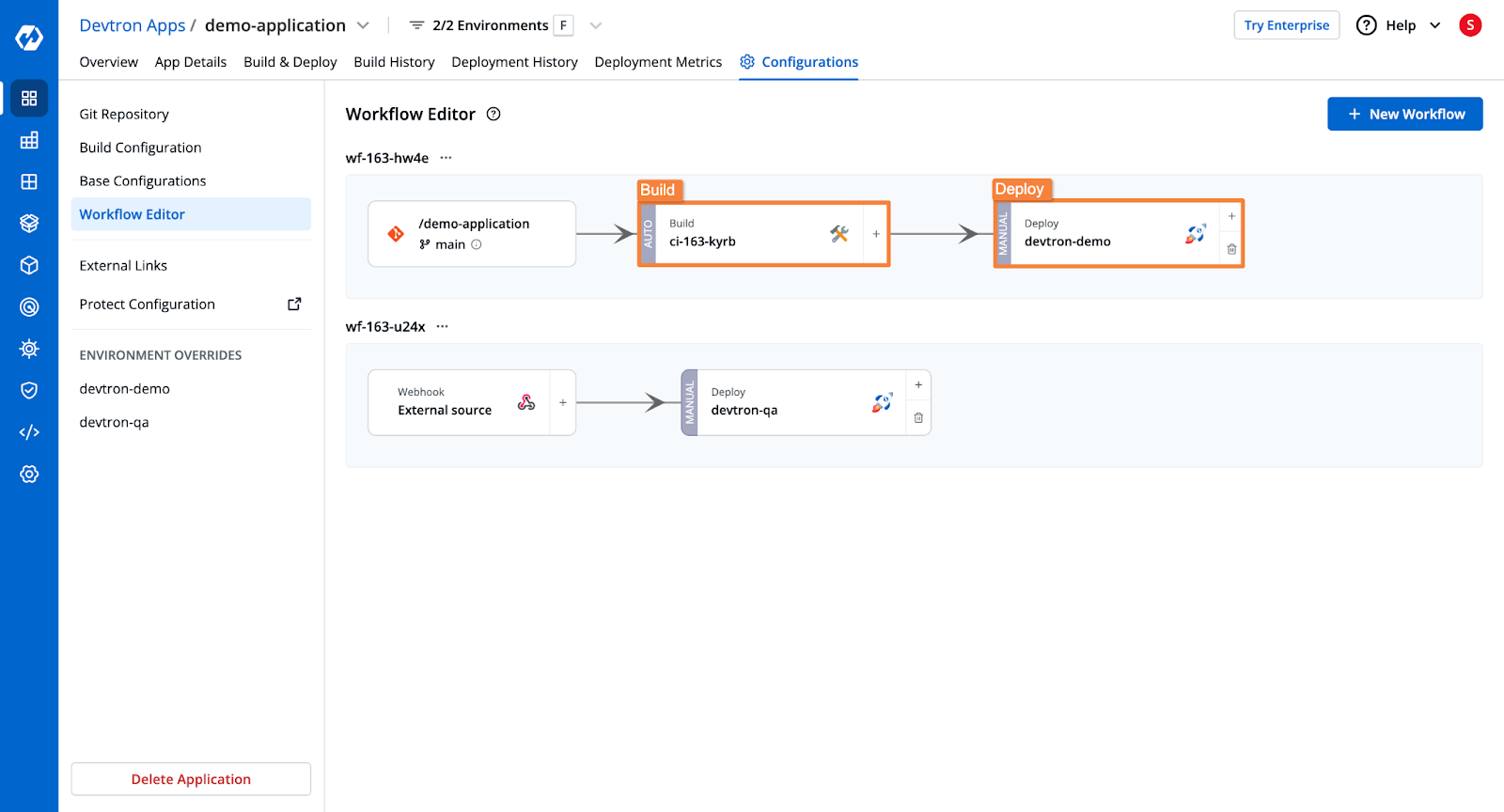
Please check the documentation to learn more about the pipeline configurations.
Step 5: Trigger the Build and Deploy Pipelines
- Select the Git branch and trigger the build stage.
- Once the build is complete, trigger the deployment stage.
- Devtron will deploy the application and show:
- Deployment status
- Application health
- Kubernetes resource details
- Security vulnerabilities
- Rollback options in case of errors
Once the application is deployed, you will be able to see the application's health, deployment status, security vulnerabilities, the Kubernetes resources of the application, and more.
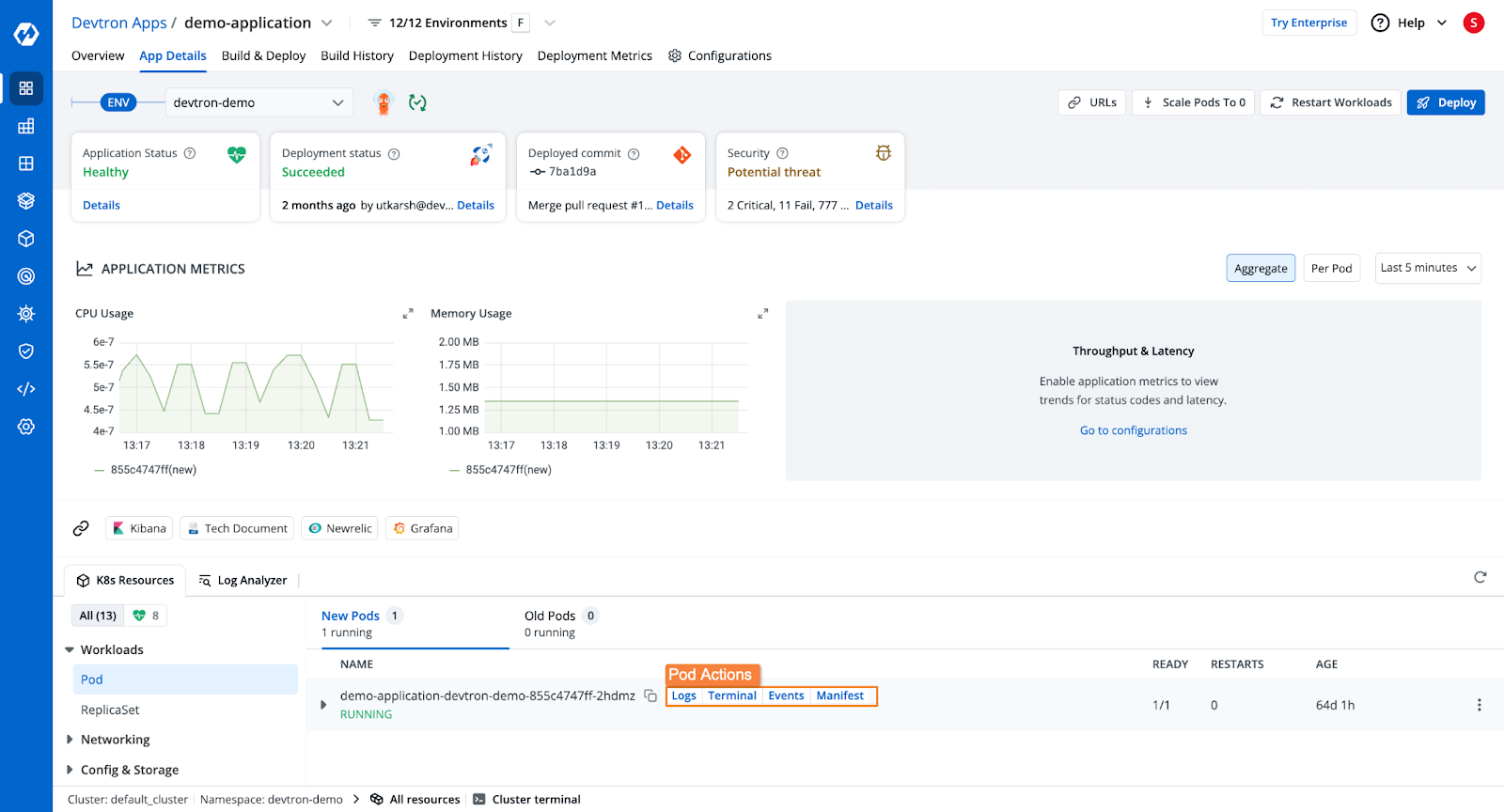
Method 2: Deploying PHP-Laravel Applications Manually Using Kubernetes
Step 1: Create the Dockerfile
A Dockerfile is a set of instructions for building a container image. Below is the Dockerfile to containerize your Express.js application:
FROM composer:1.6.5 as build
WORKDIR /app
COPY . /app
RUN composer install
FROM php:7.1.8-apache
EXPOSE 80
COPY --from=build /app /app
COPY vhost.conf /etc/apache2/sites-available/000-default.conf
RUN chown -R www-data:www-data /app \
&& a2enmod rewrite
Step 2: Build and Push the Docker Image
- Run the following command to build the Docker image:
docker build -t devtron/php-laravel-app:v1 .
- Push the image to DockerHub:
docker push devtron/php-laravel-app
Step 3: Creating the Kubernetes Deployment and Service Manifests
- Create a deployment.yaml file:
apiVersion: apps/v1
kind: Deployment
metadata:
name: laravel-kubernetes-demo
spec:
selector:
matchLabels:
run: laravel-kubernetes-demo
template:
metadata:
labels:
run: laravel-kubernetes-demo
spec:
containers:
- name: demo
image: bhushannemade/laravel-kubernetes-demo
ports:
- containerPort: 80
env:
- name: APP_KEY
value: base64:cUPmwHx4LXa4Z25HhzFiWCf7TlQmSqnt98pnuiHmzgY=
- Create a service.yaml file:
apiVersion: v1
kind: Service
metadata:
name: laravel-kubernetes-demo
spec:
type: NodePort
selector:
run: laravel-kubernetes-demo
ports:
- port: 80
targetPort: 80
nodePort: 31000
Step 4: Deploy to Kubernetes
Run the following command to apply the manifests:
kubectl apply -f deployment.yaml service.yaml
Your Express.js application has now been deployed to Kubernetes!
Common Challenges and Solutions
1. Container Image Size Management
- Use Multi-Stage Builds to separate build and runtime environments.
- Use Lightweight Base Images like Alpine or Distroless to reduce size.
2. Resource Management
- Set Memory and CPU Limits to avoid overconsumption.
- Implement Autoscaling (HPA) to handle varying workloads.
3. Deployment Strategies
- Rolling Updates to ensure zero-downtime deployments.
- Graceful Shutdown Handling to avoid breaking live traffic.
Conclusion
In this blog, we explored two approaches for deploying PHP-Laravel applications on Kubernetes:
- Manual Kubernetes Deployment using Docker and YAML manifests.
- Automated Devtron Deployment with built-in CI/CD pipelines and advanced configurations.
Using Devtron simplifies Kubernetes deployments, reducing manual efforts and improving efficiency. Start deploying applications today using Devtron’s platform!
FAQ
What are the essential prerequisites for deploying a Laravel application on Kubernetes?
You need a Laravel-PHP application, Docker, Kubectl, Helm, and a Kubernetes cluster (like Microk8s).
Why should you containerize your Laravel application?
Containerization makes your application portable, infrastructure-agnostic, and easier to scale and manage in cloud environments.
What is Kubernetes and how does it benefit Laravel applications?
Kubernetes is an open-source container orchestration platform that automates application deployment, scaling, and management. It benefits Laravel applications by providing scalability, reliability, and easy management in cloud environments.
What are Kubernetes manifests and why are they important?
Kubernetes manifests are YAML files that define the desired state of Kubernetes resources like pods, deployments, and services. They ensure proper configuration, scaling, and management of applications in a Kubernetes cluster.